As a junior developer, leveraging AI tools like large language models (LLMs) can significantly accelerate your learning and productivity. Here are 10 practical tips to help you make the most of these tools:
1. Use AI to understand unfamiliar code
When facing unfamiliar code, ask an LLM to explain it step by step. For example:
curl -X POST "https://api.openai.com/v1/chat/completions" -H "Content-Type: application/json" -H "Authorization: Bearer $OPENAI_API_KEY" -d '{"model": "gpt-4", "messages": [{"role": "user", "content": "Explain this code: function memoize(fn) { const cache = {}; return function(...args) { const key = JSON.stringify(args); if (!(key in cache)) { cache[key] = fn.apply(this, args); } return cache[key]; }}"}]}'
2. Learn to write effective prompts
Craft specific, context-rich prompts. Include:
- Your programming language/framework
- What you’ve already tried
- Error messages
- Specific requirements
3. Use AI for code reviews
Before submitting PRs, have an LLM review your code for:
- Potential bugs
- Performance issues
- Style inconsistencies
- Security vulnerabilities
4. Generate test cases
Ask AI to help create comprehensive test cases for your functions:
// Generated by AI
describe('userAuthentication', () => {
it('should return true for valid credentials', () => {
expect(userAuthentication('validUser', 'correctPassword')).toBe(true);
});
it('should return false for invalid username', () => {
expect(userAuthentication('invalidUser', 'correctPassword')).toBe(false);
});
// More test cases…
});
5. Learn concepts through examples
Ask for practical examples when learning new concepts:
“Show me 3 examples of React custom hooks with different use cases and explain how they work”
6. Use AI to refactor code
Have AI suggest improvements to your existing code:
“How can I refactor this function to be more efficient and readable?”
7. Verify AI-generated code
Always:
- Understand what the generated code does
- Test it thoroughly
- Check for edge cases
- Verify security implications
- Don’t blindly copy-paste
8. Enhance documentation skills
Use AI to help write clear documentation for your code:
/**
* Formats a date object according to the specified locale and options
*
* @param {Date} date - The date object to format
* @param {string} [locale='en-US'] - The locale to use for formatting
* @param {Object} [options] - Formatting options following Intl.DateTimeFormat specs
* @returns {string} The formatted date string
*
* @example
* // Returns "January 1, 2023"
* formatDate(new Date(2023, 0, 1))
*/
function formatDate(date, locale = 'en-US', options = { month: 'long', day: 'numeric', year: 'numeric' }) {
return new Intl.DateTimeFormat(locale, options).format(date);
}
9. Create learning roadmaps
Ask AI to create personalized learning paths:
“I want to become a full-stack developer with React and Node.js. Create a 6-month learning roadmap with weekly goals and recommended resources.”
10. Debug with AI
When stuck on a bug, provide:
- The error message
- Relevant code
- What you’ve already tried
- Expected behavior
For example: “I’m getting ‘TypeError: Cannot read property ‘map’ of undefined’ in my React component. Here’s my code: [code]. I’ve checked that the API call is successful. What could be causing this?”
Bonus tip: Balance AI use with learning
Remember that AI is a tool to enhance your learning, not replace it. Use AI to accelerate understanding, but make sure you’re developing your own problem-solving skills by:
- Understanding why solutions work
- Implementing solutions yourself after getting guidance
- Challenging yourself to solve problems before asking AI
By following these tips, you’ll be able to use AI tools effectively while continuing to grow as a developer.
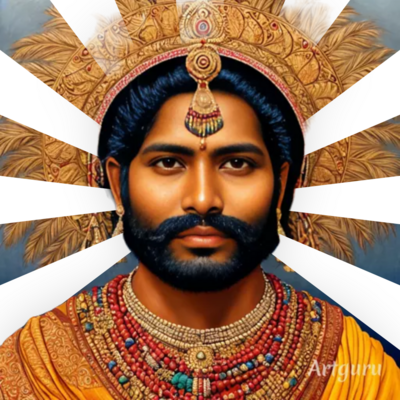
Hi, I’m Owen! I am your friendly Aussie for everything related to web development and artificial intelligence.